How to Scrape Baidu Search Results
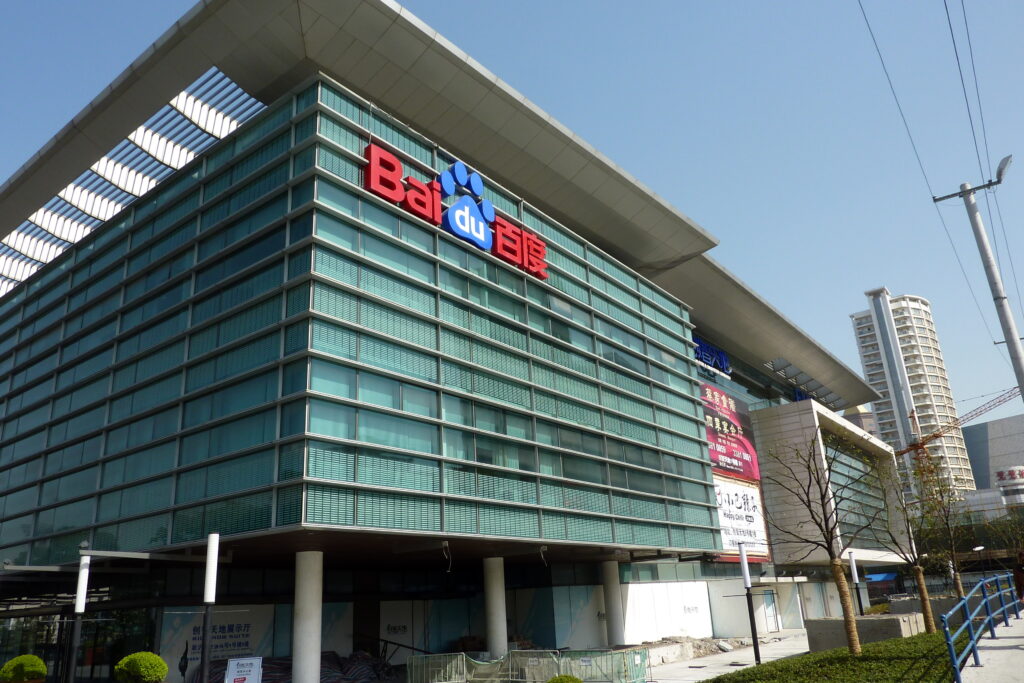
When it comes to tapping into the rich data pool of the Chinese web, Baidu stands out as a crucial resource. As China’s most widely used search engine, it offers unparalleled access to local search trends, brand visibility, and user behavior across various sectors. Whether you’re an SEO professional, a digital marketer, or a data analyst, learning how to scrape Baidu search results gives you a competitive edge. In this guide, we’ll walk you through smart strategies, practical tools, and real use cases that make the process smooth and efficient.
Scraping Baidu is not as straightforward as scraping Google due to geo-restrictions, anti-bot measures, and language-specific nuances. However, with the right proxy infrastructure, parsing tools, and smart techniques, it becomes not only feasible but scalable. You’ll also see how services like ProxyTee can play a pivotal role in powering your scraping operation with performance and anonymity.
Why Scrape Baidu Search Results
Before diving into the how, let’s understand the why. Scraping Baidu search results can deliver valuable insights for numerous business and research purposes. Some of the most common use cases include:
- Market Intelligence: Track competitor ranking, keyword trends, and user preferences in the Chinese market.
- Brand Monitoring: Keep an eye on brand mentions, sentiment, and visibility across Baidu SERPs.
- Ad Verification: Validate if and how your paid campaigns or affiliate links appear on Chinese web platforms.
- Academic or Data Research: Conduct linguistic, sociological, or behavioral studies using search trends and query suggestions.
Since Baidu operates under China’s unique internet ecosystem, automated access needs to mimic real user behavior and comply with anti-bot systems to avoid detection.
Setting Up Proxies to Access Baidu Successfully
Baidu tends to block non-Chinese IPs or repeated requests from the same IP. That’s where proxies come into play. By rotating through a large pool of residential IPs that originate from China or appear authentic, you can prevent getting blocked and maintain uninterrupted access.
Unlimited residential proxy services are ideal for this task. They provide genuine IPs from real users, helping you avoid common restrictions. With ProxyTee, you get access to 20M+ IPs with built-in Auto-Rotation, Global IP Coverage, and Multiple Protocol Support, ensuring each request looks like it comes from a different device and location.
Having Unlimited Bandwidth also ensures your scraping tasks run at scale without worrying about data caps or throttling.
Is it Legal to Scrape Baidu?
Collecting publicly available data, including Baidu’s search results, is often legal. However, ensure that your web scraper doesn’t breach any laws or collect copyrighted data. When in doubt, seek legal counsel.
Getting Started with Baidu Scraping
Before starting, make sure you have Python installed and have set up a virtual environment. This will keep the libraries you will be using separate from your main install. Here are basic commands for that, in the given order:
python -m venv env
(creates a virtual environment named “env”), or whatever name you like.source env/bin/activate
(activates the virtual environment).pip install requests
(installs the `requests` library).deactivate
to end the session in virtual env
How to Form Baidu URLs
You’ll need to construct URLs for both desktop and mobile devices to target the content you require. Baidu uses the following formats:
Desktop Devices:
https://www.baidu.<domain>/s?ie=utf-8&wd=<query>&rn=<limit>&pn=<calculated_start_page>
Mobile Devices:
https://m.baidu.<domain>/s?ie=utf-8&word=<query>&rn=<limit>&pn=<calculated_start_page>
Where:
domain
: .com for English and .cn for Chinese content.query
: The search keyword with spaces replaced by %20. Use `wd` for desktop and `word` for mobile.limit
: The number of results per page.calculated_start_page
: The starting point (calculated as `Limit * Start_page – Limit`). For example, to view the third page, which shows five items per page, use a value of 10, as 5*3 – 5 = 10
For example, to search for “nike shoes” on the fifth page, showing ten results, the URLs would be:
Desktop:
https://www.baidu.com/s?ie=utf-8&wd=nike%20shoes&rn=10&pn=40
Mobile:
https://m.baidu.com/s?ie=utf-8&word=nike%20shoes&rn=10&pn=40
Tutorial on Scraping Baidu Search Results with Python
Here is a step by step guide how to do it with Python and ProxyTee:
1️⃣ Import Necessary Libraries
import requests
from pprint import pprint
import json
2️⃣ Set your API Endpoint
Set up the URL for accessing the data through ProxyTee
url = 'https://api.proxytee.com/v1/queries'
3️⃣ Configure Authentication
Obtain your credentials (API username and password) from ProxyTee and use them as follows:
auth = ('your_api_username', 'your_api_password')
4️⃣ Create Your Payload
The `payload` dictionary holds the parameters for your search request. Modify them to fit your use case, and always point to the search query and geo-targeting. Here’s an example:
payload = {
'source': 'universal',
'url': 'https://www.baidu.com/s?ie=utf-8&wd=nike&rn=50',
'geo_location': 'United States',
'user_agent_type': 'desktop_firefox'
}
5️⃣ Send POST Request
Pass parameters in the body of the request using Python library `requests`.
response = requests.post(url, json=payload, auth=auth, timeout=180)
6️⃣ Load and Print the Data
Parse the server’s response, typically in a JSON or HTML document, for more convenient usage. The sample shows two possibilities.
json_data = response.json()
pprint(json_data)
with open('baidu.html', 'w') as f:
f.write(response.json()['results'][0]['content'])
7️⃣ Full Code Example
Here is a full snippet, which contains all steps described:
import requests
from pprint import pprint
import json
payload = {
'source': 'universal',
'url': 'https://www.baidu.com/s?ie=utf-8&wd=nike&rn=50',
'geo_location': 'United States',
'user_agent_type': 'desktop_firefox'
}
url = 'https://api.proxytee.com/v1/queries'
auth = ('your_api_username', 'your_api_password')
response = requests.post(url, json=payload, auth=auth, timeout=180)
json_data = response.json()
pprint(json_data)
with open('baidu.html', 'w') as f:
f.write(response.json()['results'][0]['content'])
Output Sample: When the code executes without any errors, you will get a status message showing 200 status code as well as a pretty JSON dump of the requested document, and additionally, a saved .html file will appear in your directory.
Real Use Cases from SEO Teams and Analysts
Let’s look at how professionals are applying these strategies in real scenarios:
- Global eCommerce Brand: A team of SEO analysts uses rotating proxies to track product-related keywords and monitor Chinese competitors on Baidu. Their setup includes auto-rotating residential IPs and a headless browser to capture SERPs for daily reports.
- Digital Ad Network: An ad verification specialist uses scraped search data to validate how and where sponsored links appear in Baidu results, confirming CTR placements and detecting unauthorized brand usage.
- Market Research Agency: Researchers collect large-scale Baidu data to analyze regional sentiment trends around public policy by extracting related keyword suggestions and ranking pages across cities.
- Startup Launching in China: A SaaS startup scrapes Baidu’s organic and paid listings to identify which local services dominate their niche, adapting their go-to-market strategy based on the findings.
These examples highlight the diversity of ways to scrape Baidu search results for competitive insights and data-driven decisions.
Handling Anti-Bot Systems with Smarter Techniques
Baidu, like most search engines, deploys rate-limiting, CAPTCHA challenges, and bot-detection algorithms. Bypassing these responsibly requires a mix of proxy strategy and user behavior simulation. Here are a few advanced tips:
- Rotate IPs and User-Agents: Change them every few requests to simulate organic traffic.
- Time Delays: Introduce random wait times between requests to avoid tripping rate limits.
- Session Persistence: Maintain cookies and local storage to replicate genuine browsing sessions.
- Captcha Handling: Use services like 2Captcha if your scraping volume is large and encountering frequent challenges.
Combining these techniques with ProxyTee’s Simple & Clean GUI can streamline your workflow, especially if managing multiple concurrent tasks.
How to Scale Baidu Scraping with ProxyTee
Scaling is where most solo setups struggle. Proxy management, concurrency control, and bandwidth handling become crucial as your data demand grows. ProxyTee allows scaling effortlessly by offering tiered Pricing plans based on port count. Each plan includes full access to residential proxies with rotation timing between 3 and 60 minutes.
You can integrate their features through API, monitor active sessions, and rotate IPs automatically to keep your Baidu scraper efficient and undetected. Whether you are scraping for SEO analysis or massive keyword research projects, a stable infrastructure like this makes a measurable difference.
Final Thoughts on How to Scrape Baidu Search Results
Being able to scrape Baidu search results reliably unlocks a world of strategic insight for businesses operating in or analyzing the Chinese market. From competitor tracking to localized SEO strategies, the possibilities are vast. The key is to use a mix of smart scraping practices, modern parsing tools, and a robust proxy network to maintain anonymity and consistency.
With solutions like ProxyTee, which provides a powerful combination of residential IPs, unlimited bandwidth, and global support, your scraper becomes future-proof. Whether you are just starting out or managing large-scale operations, having a trusted proxy provider is a must.