How to Read JSON Files in JavaScript: A ProxyTee Tutorial
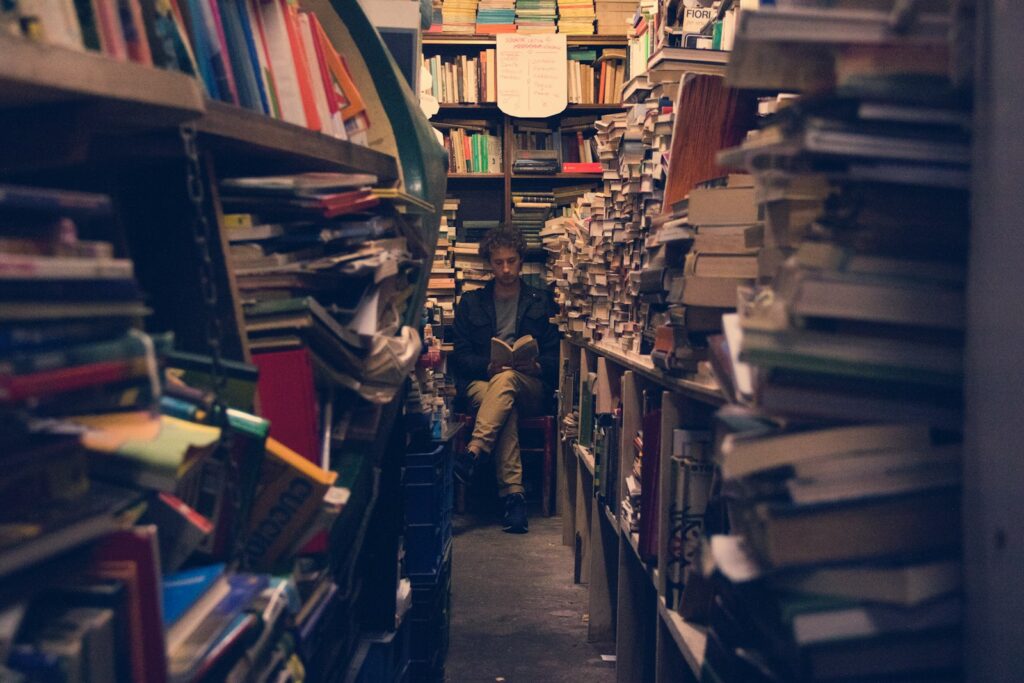
When working with servers, fetching and saving data from external sources is a common task. For these situations, a uniform format is needed that’s easy to access and read. JSON (JavaScript Object Notation) serves this purpose perfectly. It’s a lightweight format, easy to read and write, that automated systems can easily parse and generate. It’s primarily used in web applications to transmit data. Today, we’ll explore how to load and read JSON files in JavaScript, and how ProxyTee can enhance your data handling workflows.
Data Representation in JSON Files
In JSON, data is represented in (key, value) pairs. Each piece of data is identified by a unique key, and its value can be of various types, such as strings, numbers, arrays, objects, booleans, and null. Here’s a sample JSON structure:
{
"products": [
{
"id": 1,
"name": "Smartphone",
"price": 500,
"description": "A smartphone with wide-angle camera",
"stock": 30
},
{
"id": 2,
"name": "Laptop",
"price": 799,
"description": "A laptop with excellent battery life",
"stock": 20
}
]
}
Let’s see how you can load this data using JavaScript and how ProxyTee fits into your workflow.
Read JSON Data in JavaScript
We’ll discuss a few ways to load JSON data using JavaScript:
1️⃣ Using Require/Import Modules
To import JSON data, Node.js uses the require()
function. In modern ES6 JavaScript, importing a JSON file is straightforward. Let’s see the code below:
Using Node.js:
// Node.js
const data = require('./products.json');
console.log(data);
The require()
function searches for products.json
in the same directory and reads the JSON file in JavaScript object format.
Using ES6:
// Browser with a module bundler (e.g., Webpack) or modern Node.js
import data from './products.json' with { type: "json" };
console.log(data);
With ES6, remember to update your package.json
file with "type": "module"
.
2️⃣ Using Fetch API to Read JSON File
The Fetch API is used to fetch resources from the network, including JSON files. It returns a Promise that resolves to a response object. Here’s an example using a demo e-commerce website.
fetch('https://scraping-demo-api-json-server.vercel.app/products')
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok ' + response.statusText);
}
return response.json();
})
.then(data => {
displayProducts(data);
})
.catch(error => {
console.error('There has been a problem with your fetch operation:', error);
});
function displayProducts(products) {
products.forEach(product => {
console.log(`\x1b[32mProduct:\x1b[0m ${product.game_name}, \x1b[32mIn Stock:\x1b[0m ${product.inStock}`);
});
}
This code fetches the JSON data, checks for errors, and displays the product data.
3️⃣ Using the File Reader API
The FileReader API reads a local JSON file from your PC. The web application asynchronously reads the file’s contents. Here’s how it works:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Read JSON File</title>
</head>
<body>
<input type="file" id="fileInput" accept=".json" />
<div id="productList"></div>
<script>
document.getElementById("fileInput").addEventListener("change", function (event) {
const file = event.target.files[0];
if (file) {
const reader = new FileReader();
reader.onload = function (e) {
try {
const data = JSON.parse(e.target.result);
displayProducts(data.products);
} catch (error) {
console.error("Error parsing JSON:", error);
}
};
reader.readAsText(file);
}
});
function displayProducts(products) {
const productList = document.getElementById("productList");
productList.innerHTML = "";
products.forEach((product) => {
const productDiv = document.createElement("div");
productDiv.innerHTML = `Product: ${product.name}, Price: $${product.price}`;
productList.appendChild(productDiv);
});
}
</script>
</body>
</html>
This HTML code includes an input element for file selection. When you select a file, the FileReader API reads its content, parses it as JSON, and then it displays the data using the displayProducts()
function. This setup allows you to load JSON from your computer quickly in a browser. Use cases such as web scraping and processing configurations can benefit significantly from JSON integration with JavaScript and an affordable and reliable solution like ProxyTee can further improve your workflows.
ProxyTee for Enhanced Data Operations
When using JavaScript to read and process JSON data, especially for tasks like web scraping, you might need robust proxy solutions. This is where ProxyTee comes into play, enhancing your data acquisition process by offering a stable and reliable environment. ProxyTee’s Unlimited Residential Proxies provide a strong foundation, with rotating IPs, unlimited bandwidth and high levels of anonymity, and thus a much better value for your money compared to alternatives.
- Unlimited bandwidth ensures smooth data flow, essential for tasks like web scraping where the transfer of data is quite heavy.
- The global IP pool lets you access resources across various regions seamlessly, without having to manage a network by yourself.
- Auto-rotation feature rotates IPs automatically every 3 to 60 minutes, this will prevent blocks when accessing specific resources.
- Multiple Protocol Support makes sure all of your infrastructure can seamlessly integrate, offering both HTTP and SOCKS5 protocol.
Common Errors
Here are some frequent errors when reading JSON files:
- CORS Errors: These occur when retrieving JSON from a different origin. Make sure the server has the necessary headers to permit requests from different origins.
- Parsing Errors: These are due to invalid JSON formats. Always validate your JSON data.
- Security: Watch out for JSON injection attacks. Always handle your data safely and sanitize inputs.
Common Use Cases
- Web Scraping: JSON is ideal for extracting structured data from web pages. Tools that perform web scraping often parse scraped data into JSON format.
- Configuration Files: JSON is commonly used for configuration files due to its simplicity.
- Data Storage: JSON is used to store and retrieve data in file systems and databases, like NoSQL DB.
- API Integration JSON is heavily used in API communication due to the format’s simplicity.
Advanced Tips
- Performance Optimization: For large JSON files, consider streaming parsers like Node.js’ JSONStream.
- Comparison with XML: JSON’s concise structure is better for data exchange than XML, it also results in less network overhead and faster data transfer.