Build a Fast Web Scraper with Golang and ProxyTee in 2025
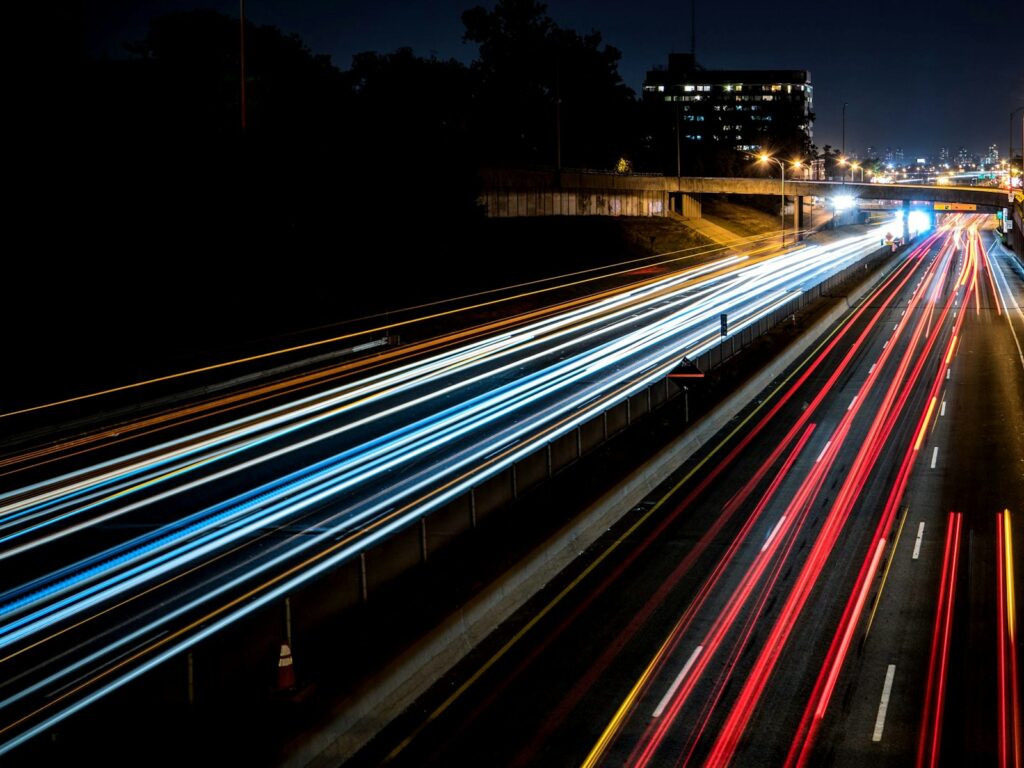
Web scraping is the automated process of extracting data from websites. It’s a crucial tool for gathering information, and often, the efficiency of a scraper is as important as the data it retrieves. While many tutorials focus on popular languages like Python and JavaScript, this post dives into how to build a fast and efficient web scraper using Golang, enhanced with ProxyTee for reliable IP rotation and anonymity.
Why Choose Golang for Web Scraping?
Golang, or Go, is engineered to blend the performance of C with the ease of use of Python and JavaScript. It’s particularly strong in networking and multiprocessing, making it an excellent choice for web scraping. Go’s concurrency features enable it to handle multiple tasks simultaneously, resulting in faster scraping times, which is beneficial when processing large datasets. Using ProxyTee’s residential proxies with Go ensures that your scraping operations are also robust and less likely to be blocked by target websites.
Getting Started with Golang
Before diving into code, you’ll need to set up Go on your machine. Here’s a brief overview of how to do that:
- Install Go: Download the appropriate installer from the official Go downloads page. This site provides installers for Windows, macOS, and Linux.
- Set Up Your Environment: You’ll want to use a code editor or an IDE that supports Go. Visual Studio Code (VS Code) is a great option, along with the Go extension for easy development.
Using Colly for Web Scraping
Colly is a fast, popular web scraping framework for Go that simplifies the process of creating crawlers, scrapers, and spiders. It manages cookies, sessions, and supports caching and `robots.txt`. For our tutorial, we’ll use Colly to extract data from `books.toscrape.com`, a sample website for scraping practices. Here’s how to integrate it:
- Import Packages: In your Go file, you’ll use the `import` directive to include necessary packages, including Colly (`github.com/gocolly/colly`).
- Set Up Your Project: Start a new Go module and then use the `go get` command to install Colly, which will manage dependencies and create `go.mod` and `go.sum` files.
For example:
go mod init proxytee.com/web-scraper-go
go get github.com/gocolly/colly
Implementing a Web Scraper with ProxyTee and Colly
Once Colly is set up, you can start implementing the web scraping logic. Below is an example of how you can combine Colly with ProxyTee to get robust and reliable data:
- Set Up Event Handlers: Use the `OnRequest`, `OnResponse`, and `OnHTML` events to handle the scraping workflow. For example, you’ll use `OnHTML` to target specific elements by their CSS selectors (e.g., ‘.product_pod’ for a list of books).
- Using ProxyTee Auto Rotation: Take advantage of ProxyTee’s auto-rotation feature to change IPs at intervals from 3 to 60 minutes to prevent website detection of your scrapers. This feature can be configured to best fit the scraping task.
1️⃣ Handle Pagination: If needed, locate the “next” button on a page (e.g., with the CSS selector `.next > a`) and use `Visit()` to crawl subsequent pages.
c.OnHTML(".next a", func(e *colly.HTMLElement) {
nextPage := e.Attr("href")
c.Visit(e.Request.AbsoluteURL(nextPage))
})
2️⃣ Extract and Store Data: Once elements are located with selectors, you can extract the necessary data (like book titles and prices) and use ProxyTee API to save it in formats like CSV or JSON. The following code snippet explains this process:
c.OnHTML(".product_pod", func(e *colly.HTMLElement) {
book := Book{}
book.Title = e.ChildAttr(".image_container img", "alt")
book.Price = e.ChildText(".price_color")
row := []string{book.Title, book.Price}
writer.Write(row)
})
3️⃣ Integrate ProxyTee: Integrate ProxyTee to handle IP rotation and bypass website restrictions by setting proxy url into Colly.
proxyUrl := fmt.Sprintf("http://customer-%s:%[email protected]:7777", proxyUsername, proxyPassword)
c.SetProxy(proxyUrl)
4️⃣ Configure the Collector: A collector manages requests and traverses HTML pages.
c := colly.NewCollector(
colly.AllowedDomains("books.toscrape.com"),
)
Managing Your Scraping Schedule
For periodic scraping, you can integrate a scheduling package like GoCron, which lets you schedule your scraping tasks at specified times or intervals. You will install it via command:
go get github.com/go-co-op/gocron
The below code shows a simple configuration to start scrape with frequency:
my_scheduler := gocron.NewScheduler(time.UTC)
my_scheduler.Every(2).Minute().Do(BooksScraper)
my_scheduler.StartBlocking()
Alternative Golang Libraries for Web Scraping
Go offers several frameworks beyond Colly that can assist in web scraping, including:
- Ferret: A fast, portable framework focused on declarative queries to extract data.
- Gocrawl: Allows complete control of web visits, inspections, and queries via `goquery`.
- Soup: A smaller library suitable for implementing Go web scrapers with a content retrieval and parsing API.
- Hakrawler: A basic crawler good for extracting URLs and JavaScript locations.
- GoQuery: Provides functionality similar to jQuery, which is ideal for DOM manipulation during parsing.
The Ultimate Duo for Scraping: Golang and ProxyTee
Golang’s efficiency and strong concurrency features, combined with the reliability of ProxyTee’s rotating residential proxies, make it a fantastic choice for robust web scraping in 2025. By utilizing Go along with frameworks such as Colly, you can develop quick, stable, and effective scraping solutions for various data acquisition tasks.
Whether you’re new to web scraping or looking to upgrade your tech stack, Go with ProxyTee is a valuable and fast combination. With Unlimited Residential Proxies from ProxyTee, you are ready to tackle complex scraping challenges by leveraging features such as: unlimited bandwidth, global IP pool, multiple protocol, and easy-to-use interface. It’s also cheaper compared to competitors by up to 50% while delivering high quality and reliability for data acquisition.